IDEA中lombok插件的使用(可省略get/set方法)
1.安装插件
在File-Setting-Plugins-Browse Repostitories中搜索Lombok Plugin插件安装
安装完成先别急着重启,继续设置,在File-Setting-Build, Execution, Deployment-Compiler-Annotation Processors中点击Enable annotation processors
确定后重启idea
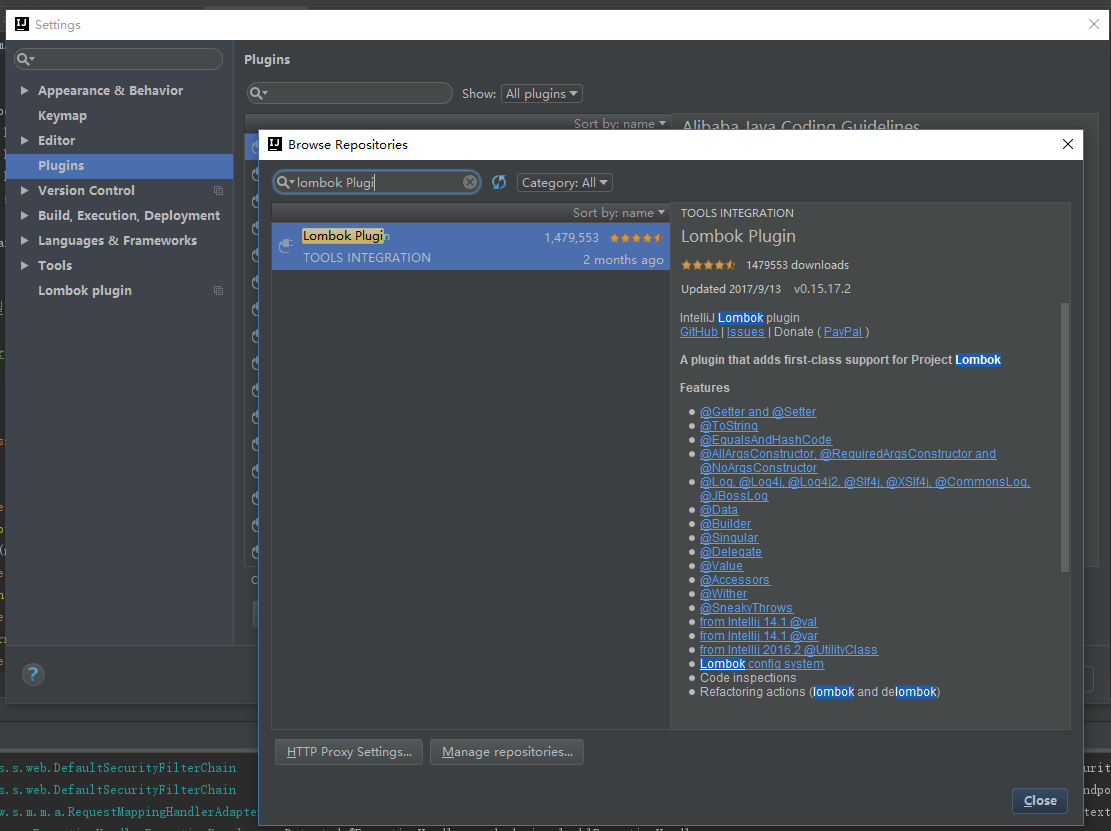
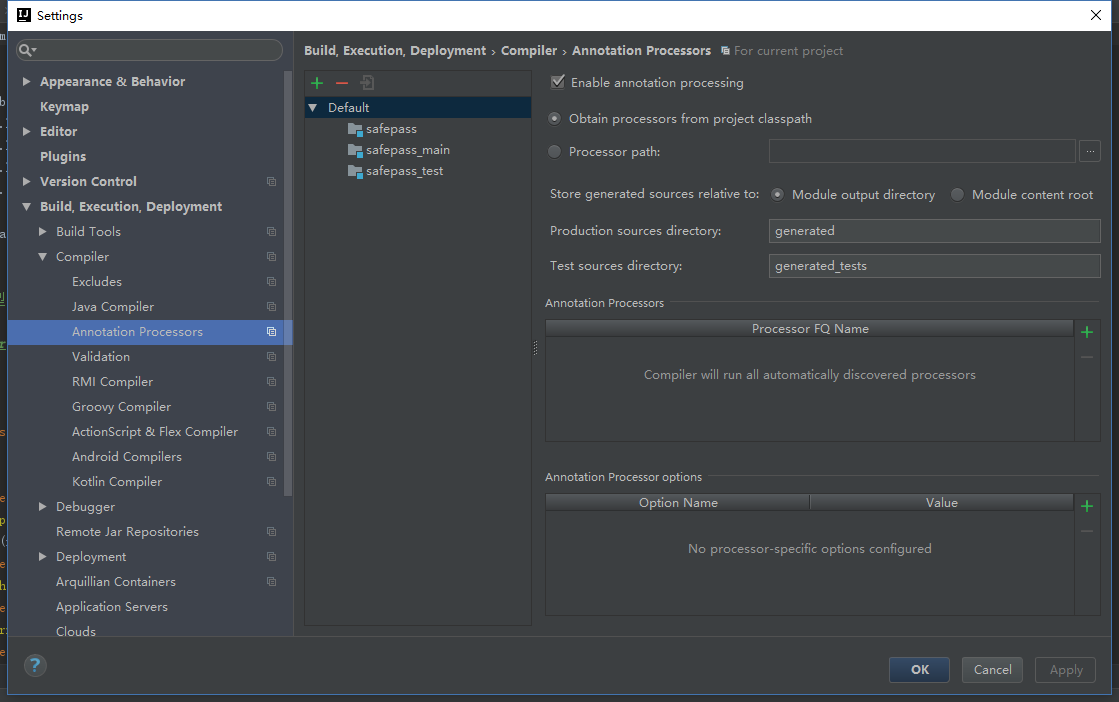
2.导入包
在maven中导入依赖
1 2 3 4 5 6
| <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <version>1.16.18</version> <scope>provided</scope> </dependency>
|
3.使用
只需要在相关类、变量上加上注解即可
常用的有
1 2 3 4 5 6
| @Data : @Setter: @Getter: @Log4j : @NoArgsConstructor: @AllArgsConstructor:
|
比如:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56
| @Entity @DynamicUpdate public class ProductCategory { @Id @GeneratedValue private Integer categoryId; private String categoryName; private Integer categoryType; public ProductCategory() { } private Date createTime; private Date updateTime; public Integer getCategoryId() { return categoryId; } public void setCategoryId(Integer categoryId) { this.categoryId = categoryId; } public String getCategoryName() { return categoryName; } public void setCategoryName(String categoryName) { this.categoryName = categoryName; } public Integer getCategoryType() { return categoryType; } public void setCategoryType(Integer categoryType) { this.categoryType = categoryType; } public Date getCreateTime() { return createTime; } public void setCreateTime(Date createTime) { this.createTime = createTime; } public Date getUpdateTime() { return updateTime; } public void setUpdateTime(Date updateTime) { this.updateTime = updateTime; } @Override public String toString() { return "ProductCategory{" + "categoryId=" + categoryId + ", categoryName='" + categoryName + '\'' + ", categoryType=" + categoryType + '}'; } }
|
在安装了 lombok 插件和 导入了 lombok 依赖后
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| @Entity @DynamicUpdate @Data public class ProductCategory { @Id @GeneratedValue private Integer categoryId; private String categoryName; private Integer categoryType; private Date createTime; private Date updateTime; public ProductCategory() { } }
|